Interim API documentation
While we build permanent documentation for our API, this document contains information on our main endpoints.
You'll see listed all of the APIs that we use to build SweetProcess. Historically these have not been made known to customers, but we are changing that starting with this document.
sweetprocess.com/accounts/tokens/
Create your secret token and include it in the Authorization header to the SweetProcess API. For example:
Provides a paginated list of procedures that the current user has access to.
Filters
team_id - filter to procedures within the given team
search - search for a procedure
tag - procedures with the given tag(s) separated by comma
policy_id - procedures with the attached policy
visible_to_user - procedures that you can see and the requested user can see
ordering - rank,name,modified_at,approved_at,last_review_at add a minus symbol to reverse the order (e.g. -rank is required for ordering search relevance)
Example using some filters
https://www.sweetprocess.com/api/v1/procedures/?tag=accounts,tax&team_id=200000&search=quarterly&ordering=-rank
Each filter is separated by an ampersand (&) and the start of the filters is indicated with a question mark (?). You should not use the same filter twice e.g. search=holidays&search=christmas as the second would overwrite the first.
Provides a paginated list of taskinstances - you must use filters to find what you are looking for. A taskinstance is a specific task run at a specific time. Compare this with a Task Template which describes a series of recurring tasks (how often it recurs etc).
Why can't I see tasks that I think I should be able to see?
Our backend will automatically filter tasks to the ones you have permission to see. This is tasks that were assigned to you or by you to someone else.
However we have an option that your account owner can turn on. It will allow teammates to see co-worker's tasks if they have permission to see the procedure/process assigned. Your account owner can find this setting in the account overview area:
Teammates can see all tasks for documents they have permission to see. Even if they were not assigned to the task.
local.sweetprocess.com/accounts/overview/
Why am I being shown very old tasks? Where are all the new ones?
You must filter by date. Please use both the due__gte (due date greater than or equal to) and due__lte (due date less than or equal to) to filter your results. This will ensure the API is returning the date range that you want to see. Otherwise the default would be to show you oldest to newest. We can't sort by newest to oldest because some tasks don't end and there would be tasks due in the year 9999 being returned!
Filters
template_id - just taskinstances belonging to the given task template
user - tasks assigned to this user (use the user's api url e.g. /api/v1/users/3/)
content_type/object_id - filter for a particular document e.g. content_type=procedure&object_id=23
completed - true/false filter for completed task instances
due__lte / due__gte - taskinstances with a due date between these two dates. This might be the most useful filter. The date should be in iso8601 format 'YYYY-MM-DDTHH:MM:SSZ'. due__lte stands for due date less than or equal to, due__gte stands for due date greater than or equal to
example using some filters
https://www.sweetprocess.com/api/v1/taskinstances/?completed=false&due__gte=2020-01-01T00:00:00Z&due__lte=2020-02-01T00:00:00Z
example fetching previous month if this is May 2025 (fetching April 2025)
https://www.sweetprocess.com/api/v1/taskinstances/?due__gte=2025-04-01T00:00:00Z&due__lte=2025-05-01T00:00:00Z
the important parts are due__gte and due__lte. I've made sure the date is greater than April 1st 2025 and less than May 1st 2025. This would give me all the results for April 2025
The first plural version can be used for
POST -> Inviting a new teammate to your account
PATCH -> changing some aspect of a teammate on your account (is_super_manager, is_super_teammate, is_billing_admin, email, name)
DELETE -> Remove the teammate from your account
The singular version is for your own logged-in account (for whoever is making the API request).
It only accepts PATCH requests. At the moment the only change you can make is for navigation_links to change the ordering of your SweetProcess dashboard navigation links.
Filters
team_id / exclude_team_id - show only teammates that are members of these teams
id / exclude_id - show only teammates matching (or not matching) these ids
status - tfa_disabled | pending_invitation | invalid_email | email_verified | no_account_access | is_guest_until, show teammates matching one of these statuses
Example inviting new teammate
sweetprocess.com/api/v1/invitations/
This API requires a POST to create a new invitation between the member and the team.
example adding a member to a team:
You can remove that member from the team too:
you will need the teamusers API
sweetprocess.com/api/v1/teamusers/
This endpoint requires a DELETE on the particular member you would like to remove from the team. for example:
If you simply request the user detail endpoint for the member that you want to delete, you'll find the information in the 'teammemberships' section:
sweetprocess.com/api/v1/users/32010/
returns a response looking like this:
Where can I kick the tires?
visit sweetprocess.com/api/v1/ (whilst logged in to your SweetProcess account)You'll see listed all of the APIs that we use to build SweetProcess. Historically these have not been made known to customers, but we are changing that starting with this document.
How would I authenticate with the API
You may use a token in the Authorization header of your request to the SweetProcess API. To get a token, visitsweetprocess.com/accounts/tokens/
Create your secret token and include it in the Authorization header to the SweetProcess API. For example:
Authorization: Token 9944b09199c62bcf9418ad846dd0e4bbdfc6ee4bHere is an example using the curl program
curl -H 'Authorization: Token 9944b09199c62bcf9418ad846dd0e4bbdfc6ee4b' https://www.sweetprocess.com/api/v1/procedures/And another example using the Python programming language (and using the 3rd party requests library)
import requests response = requests.get( 'https://www.sweetprocess.com/api/v1/procedures/', headers={'Authorization': 'Token 9944b09199c62bcf9418ad846dd0e4bbdfc6ee4b'} ) print(response.json())
Procedures
sweetprocess.com/api/v1/procedures/Provides a paginated list of procedures that the current user has access to.
Filters
team_id - filter to procedures within the given team
search - search for a procedure
tag - procedures with the given tag(s) separated by comma
policy_id - procedures with the attached policy
visible_to_user - procedures that you can see and the requested user can see
ordering - rank,name,modified_at,approved_at,last_review_at add a minus symbol to reverse the order (e.g. -rank is required for ordering search relevance)
Example using some filters
https://www.sweetprocess.com/api/v1/procedures/?tag=accounts,tax&team_id=200000&search=quarterly&ordering=-rank
Each filter is separated by an ampersand (&) and the start of the filters is indicated with a question mark (?). You should not use the same filter twice e.g. search=holidays&search=christmas as the second would overwrite the first.
Taskinstances
sweetprocess.com/api/v1/taskinstances/Provides a paginated list of taskinstances - you must use filters to find what you are looking for. A taskinstance is a specific task run at a specific time. Compare this with a Task Template which describes a series of recurring tasks (how often it recurs etc).
Why can't I see tasks that I think I should be able to see?
Our backend will automatically filter tasks to the ones you have permission to see. This is tasks that were assigned to you or by you to someone else.
However we have an option that your account owner can turn on. It will allow teammates to see co-worker's tasks if they have permission to see the procedure/process assigned. Your account owner can find this setting in the account overview area:
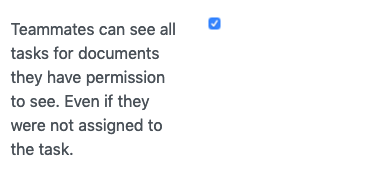
local.sweetprocess.com/accounts/overview/
Why am I being shown very old tasks? Where are all the new ones?
You must filter by date. Please use both the due__gte (due date greater than or equal to) and due__lte (due date less than or equal to) to filter your results. This will ensure the API is returning the date range that you want to see. Otherwise the default would be to show you oldest to newest. We can't sort by newest to oldest because some tasks don't end and there would be tasks due in the year 9999 being returned!
Filters
template_id - just taskinstances belonging to the given task template
user - tasks assigned to this user (use the user's api url e.g. /api/v1/users/3/)
content_type/object_id - filter for a particular document e.g. content_type=procedure&object_id=23
completed - true/false filter for completed task instances
due__lte / due__gte - taskinstances with a due date between these two dates. This might be the most useful filter. The date should be in iso8601 format 'YYYY-MM-DDTHH:MM:SSZ'. due__lte stands for due date less than or equal to, due__gte stands for due date greater than or equal to
example using some filters
https://www.sweetprocess.com/api/v1/taskinstances/?completed=false&due__gte=2020-01-01T00:00:00Z&due__lte=2020-02-01T00:00:00Z
example fetching previous month if this is May 2025 (fetching April 2025)
https://www.sweetprocess.com/api/v1/taskinstances/?due__gte=2025-04-01T00:00:00Z&due__lte=2025-05-01T00:00:00Z
the important parts are due__gte and due__lte. I've made sure the date is greater than April 1st 2025 and less than May 1st 2025. This would give me all the results for April 2025
User endpoints
We have two endpoints for interacting with teammates on your SweetProcess account.The first plural version can be used for
POST -> Inviting a new teammate to your account
PATCH -> changing some aspect of a teammate on your account (is_super_manager, is_super_teammate, is_billing_admin, email, name)
DELETE -> Remove the teammate from your account
The singular version is for your own logged-in account (for whoever is making the API request).
It only accepts PATCH requests. At the moment the only change you can make is for navigation_links to change the ordering of your SweetProcess dashboard navigation links.
Filters
team_id / exclude_team_id - show only teammates that are members of these teams
id / exclude_id - show only teammates matching (or not matching) these ids
status - tfa_disabled | pending_invitation | invalid_email | email_verified | no_account_access | is_guest_until, show teammates matching one of these statuses
Example inviting new teammate
curl https://www.sweetprocess.com/api/v1/users/ -H 'Authorization: Token 9944b09199c62bcf9418ad846dd0e4bbdfc6ee4b' -H 'Content-Type: application/json' --data '{"name": "Jake Weary", "email": "jake@example.com", "is_super_manager": 0}'
User Management
You can invite members to teams using the invitation API.sweetprocess.com/api/v1/invitations/
This API requires a POST to create a new invitation between the member and the team.
example adding a member to a team:
curl https://www.sweetprocess.com/api/v1/invitations/ -H 'Authorization: Token 9944b09199c62bcf9418ad846dd0e4bbdfc6ee4b' -H 'Content-Type: application/json' --data '[{"send_mail":false,"content_type":"team","permission":"view","object_id":118482,"to_user_id":"https://www.sweetprocess.com/api/v1/users/32010/"}]'This would add an existing member (with the url-id below)
https://www.sweetprocess.com/api/v1/users/32010/to the team with id 118482
You can remove that member from the team too:
you will need the teamusers API
sweetprocess.com/api/v1/teamusers/
This endpoint requires a DELETE on the particular member you would like to remove from the team. for example:
curl 'https://www.sweetprocess.com/api/v1/teamusers/4059787/' -X DELETE -H 'Authorization: Token 9944b09199c62bcf9418ad846dd0e4bbdfc6ee4b'Where can I find that specific teamuser URL to DELETE?
If you simply request the user detail endpoint for the member that you want to delete, you'll find the information in the 'teammemberships' section:
sweetprocess.com/api/v1/users/32010/
returns a response looking like this:
{"url":"https://www.sweetprocess.com/api/v1/users/32010/","content_type":"user","id":32010,"team_memberships":[{"url":"https://www.sweetprocess.com/api/v1/teamusers/598720/","team":"https://www.sweetprocess.com/api/v2/teams/55667/","is_manager":false}], [...]}
Did this answer your question?
If you still have a question, we’re here to help. Contact us